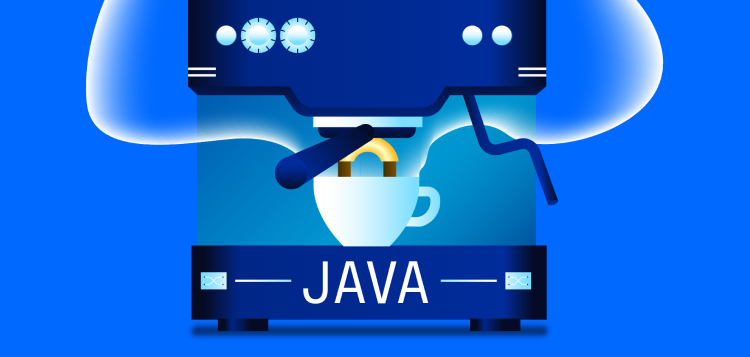
Debugging is an essential part of software development. As Java programs become increasingly complex, effective debugging tools and strategies are critical for efficiently identifying and fixing issues. This comprehensive guide will explore the key Java debugging tools available and outline core debugging strategies to apply during the development process.
Understanding Java Errors and Exceptions
Before delving into specific tools and techniques, it’s important to understand the basic types of issues that manifest in Java code. Broadly, these fall into two categories – errors and exceptions.
Errors in Java occur when the code fails to compile. Syntax mistakes cause these invalid arguments to be passed to methods, incompatible types, and other problems. Errors must be fixed for the application to run at all.
Exceptions, on the other hand, occur during execution or runtime. These are triggered when an abnormal condition arises that disrupts the normal flow of the program. Exceptions can arise due to problems like divided-by-zero errors, null pointer exceptions, out-of-bounds issues, and much more.
Hire Java developers who have a strong grasp of possible Java errors and exception scenarios to achieve effective debugging solutions when such issues occur.
Types of Java Debugging Tools
Several versatile debugging tools are available for Java, both free and paid. These range from basic logging utilities to advanced IDE integrations and profiling suites.
Some key options include:
- Logging Frameworks – Popular libraries like log4j and java.util.logging allows printing contextual debug messages from code. These logs provide visibility into the flow and key variable values.
- IDE Debuggers – Integrated debugging options in IDEs like Eclipse, IntelliJ IDEA, and NetBeans allow stepping through code, inspecting variables, setting breakpoints, hot-swapping code changes, and more.
- Profilers – Dedicated profiling tools like VisualVM, YourKit, and JProfiler help monitor and analyze application performance and memory usage during execution.
- Java Flight Recorder – An advanced profiling tool built into Oracle JDK distributions to collect diagnostic and profiling data with low overhead.
- Debugger User Interfaces – Graphical frontend interfaces like Java Debug Wire Protocol provide remote debugging for cloud platforms and containerized environments.
- Hybrid Tools – Some tools combine debugging, profiling, monitoring, and more in a single integrated suite – for example, Cisco AppDynamics and New Relic.
Popular Java Debugging Tools
Now that we know the different types of Java debugging tools available let’s look at some of the most popular debugging tools every skilled Java developer must know about. Here are some of the most widely used debugging tools for Java applications –
- Log4j
Open-source logging utility that allows printing debug messages to console or files configured at different log levels like INFO, DEBUG, ERROR, etc. Useful for tracing execution flow.
- Java Logging API
Part of core Java SDK provides logger configuration, log record creation, transport via XML/JSON/console, etc. Lightweight and simple debugging solution.
- Java Debug Wire Protocol (JDWP)
Protocol and API used for communication between JVM and Java debuggers. Enables remote debugging for cloud platforms like AWS Lambda.
- Dump Analyzer Tool (MAT)
Heap dump analyzer to check memory usage and allocation hotspots during execution to optimize garbage collector, large objects, and leaks.
- VisualVM
Visual tool combining runtime application monitoring, profiling, and CPU/memory/thread analysis into one interface. Easy integration with JDK.
- Java Flight Recorder
Robust low overhead profiler fully integrated with HotSpot JVM. Enables gathering key metrics around code performance, memory, threading, etc, without restart.
- Java Mission Control
An advanced tool from Oracle extending JDK profiling and monitoring capabilities via plugins and graphical interfaces. Analyze HotSpot JVM internals.
- YourKit Java Profiler
Leading commercial Java profiler supporting CPU, memory, thread, and lock tracking with minimal overhead. Integrates with major Java app servers.
- Splunk
Analytics platform to aggregate, analyze, and visualize outputs from Java stack – logs, metrics, apps, and services. Great for monitoring end-to-end transactions.
Key Debugging Strategies
In addition to understanding the available tooling, software developers need an effective debugging process to systematically identify and resolve bugs as quickly as possible. Here are some core debugging strategies for Java:
1. Reproduce the Error
The first step should always be attempting to reproduce the bug through deliberate actions in code. This will offer additional context and help confirm if the issue is demonstrated consistently or only under certain conditions. Verifying reproducibility also eliminates intermittent bugs or false positives.
2. Log and Print Debug Lines
Adding strategic print statements is one easy way to follow code execution flow and check if variables change as expected. Logging frameworks extend this concept further for capturing debug messages right from code. Leveraging logging effectively is key for gaining visibility into complex processes.
3. Use Breakpoints while Debugging
Most Java IDEs allow setting breakpoints that pause execution to enable stepping through code line by line. This is extremely useful for inspecting stack call sequences and verifying program state as variables change. Fully leveraging breakpoints is critical for effective debugging cycles.
4. Understand Exception Traces
In languages like Java, exceptions bubble up until caught and leave behind full stack traces. Analyzing these traces helps uncover the originating spot and path followed by the exception as it propagates upwards. The clues present in the stack trace can quickly pinpoint unexpected control flows.
5. Profile Performance Hotspots
Runtime issues like slowness, hanging, and excessive resource usage should be examined via profiling tools. Java profilers can capture metrics to measure memory allocation, processor time, database load, network I/O, etc. This data exposes performance hotspots for targeted optimization.
6. Check Log Messages
Debug or error messages emitted by the runtime environment and application logs contain invaluable context for common issues. For server-based Java apps, analyzing logs via platforms like Splunk is key for rapid diagnosis. Pay close attention to repeated messages and error trends.
7. Monitor Transactions End-to-End
Following end-to-end transaction flows across microservices, messaging systems, databases, caches, and other application layers provides visibility into failures. Debugging only narrow slices can miss bigger interconnection issues. Adopt monitoring to track flows holistically.
8. Refactor Code
Sometimes, the code structure hinders diagnosability by having too many control flows, excessive branching, shotgun surgery code changes, etc. Refactoring code to limit complexity while keeping good test coverage yields easier debugging.
9. Verify Input Data
Bad input data is a common cause of exceptions and failures. Code that makes faulty assumptions about data can lead to uncaught edge cases. Defensively checking input data and adding guards prevents assumptions from turning into bugs.
10. Add Instrumentation Code
Inserting temporary instrumentation code blocks during debugging – like pre/post condition parameter checks, mock services, fault injections, etc. is a technique to validate assumptions and uncover blind spots. This instrumentation helps crystallize diagnosis.
To identify such solutions, it is advisable to leverage IT strategy & consulting services from a reliable IT company with experience in Java development.
Key Challenges with Debugging Java
While a wide range of tooling makes Java debugging manageable in most cases, developers still face some key challenges:
Complex failure
Large Java applications have distributed services, libraries, frameworks, and container layers. This diversity makes narrowing down the root causes difficult.
Intermittent issues:
Bugs that cannot be reliably reproduced are extremely hard to fix with testing alone.
Resource constraints:
Debugging performance issues related to memory, IO, sockets, database, etc., requires capturing metrics from prod systems.
Dependency conflicts:
Conflicts between library versions used by internal teams can trigger unexpected runtime issues not seen during development.
Production access limits:
Due to change control processes and security protocols, debugging capabilities are usually restricted on live production servers.
Overcoming these requires adopting holistic monitoring, smart logging, and reference architectures for smoother bug diagnosis across diverse systems.
To conclude the discussion around debugging practices, the following are some key tips for effective and optimized debugging cycles during Java application development:
- Define expected vs actual behavior diagnosis.
- Break down complex processes into smaller isolated units for targeted debugging.
- Search through open issue trackers for potential existing diagnosis clues.
- Strictly minimize the variables in play by temporarily stubbing out irrelevant modules.
- Validate assumed direct correlations – consider all potential factors contributing to the issue.
- Resist the temptation to dive into debugging non-reproducible issues.
- Set up logging levels judiciously – avoid verbose noise during initial passes.
- Leverage IDE capabilities aggressively for step debugging and hot-swapping where possible.
- Take notes on insights gained during each debugging attempt to correlate learnings.
- Objectively quantify improvements across iterations – code changes, metric impacts, etc.
Final Words
The complexity and scale of Java applications make access to versatile debugging tooling critical for engineers. Having visibility into code execution flows, system resource monitoring, log analysis, and automated crash reporting is key to diagnosing issues faster during development and production. With the right set of debugging tools aligned to the environment and metrics-driven troubleshooting workflows, engineers can effectively tackle a diverse range of Java issues.
Tech World Times (TWT), a global collective focusing on the latest tech news and trends in blockchain, Fintech, Development & Testing, AI and Startups. If you are looking for the guest post then contact at techworldtimes@gmail.com